SpeakOut! includes a number of webhooks to enable you to extend the functionality. The hook name should be adequate description of the trigger. Webhooks are disabled by default and can be enabled in the security tab of SpeakOut! settings.
This is not meant to be a guide on how to use webhooks, but briefly, on certain actions you can add your own code to the theme’s functions.php file to do something. e.g. after a petition is signed you could send an email to your self or add it to a different database or update a mailing list or whatever you can come up with.
speakout_after_petition_signed
Triggered after petition is signed with no check on whether it is to be confirmed or whether petition is sent. All signature data are passed as an array.
script: /includes/class.signature.php
array values: petitions_id, honorific, first_name, last_name, email, date, confirmation_code, is_confirmed, optin, street_address, city, state, postcode, country, custom_field, custom_field2, custom_field3, custom_field4, custom_field5, custom_field6, custom_field7, custom_message, language, IP_address, anonymise
Example:
add_action("speakout_after_petition_signed", "send_signature_notification", 10);
function send_signature_notification( $data ){
mail("administrator@example.com",
"Notification from SpeakOut! plugin",
"Petition ID " . $data["petitions_id"] . " has been signed" . "\r\n" .
"Name: " .$data["honorific"] . " " . $data["first_name"]. " " . $data["last_name"] . "\r\n" .
"Email: " . $data["email"] . "\r\n" .
"Optin: " . $data["optin"] . "\r\n" .
"Custom field 1: " . $data["custom_field"],""
);}
Result:
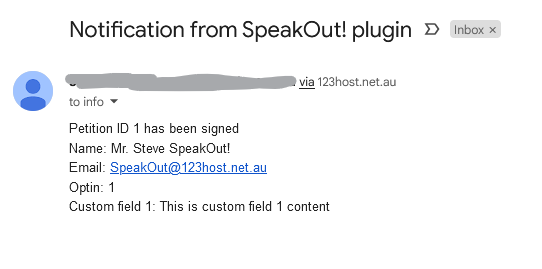
speakout_after_confirmation_sent
Triggered when someone signs and the confirmation email is sent to them
script: /includes/class.mail.php
values: petition id, email address, petition title
Example:
add_action("speakout_after_confirmation_sent", "send_confirmation_notification", 10, 3);
function send_confirmation_notification( $id, $email, $title ){
mail("administrator@example.com",
"Notification from SpeakOut! plugin", $email . " has just been sent a confirmation email for petition " . $id . " - " . $title );""
);}
Result:
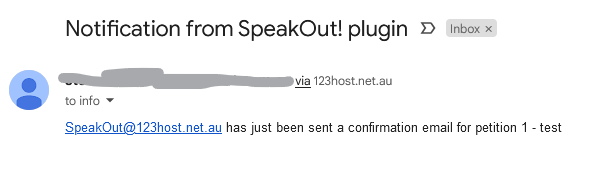
speakout_signature_confirmed
Triggered after signer successfully confirms email address
script: /includes/confirmations.php
values: id, title, email, first name, last name, Active Campaign list ID, Mailchimp List ID, Mailerlite group ID, Sendy list ID
Example:
add_action("speakout_signature_confirmed", "update_mailing_list", 10, 9);
function update_mailing_list( $id, $title, $email, $firstName, $lastName, $activecampaignList, $mailchimpList, $mailerliteGroup, $sendyList ){
//create an array including required data
$data = array( 'petitionID' => $id, 'email' => $email, 'listID' => $mailchimpList);
//insert it into database
$wpdb->insert( 'another_table', $data );
}
speakout_after_petition_sent
Triggered when petition is sent. This is distinct from when someone signs or confirms as you may have the option to only collect emails enabled.
script: /includes/class.mail.php
values: petition id, petition title, doBCC?, from address (the signer), target email address(es), email subject, email message
Example:
add_action("speakout_after_petition_sent", "thank_team", 10, 7);
function thank_team( $id, $title, $doBCC, $from, $email, $subject, $email_message ){
mail("team@example.com",
"Great work team", "We have just had another '" . $title . "' petition sent. Keep up the good work promoting this campaign."
);
}
Result:
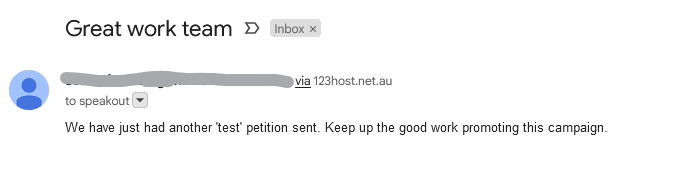
speakout_signature_confirmed
Triggered after signer successfully confirms email address. Note that in the free version the mailing lists, and therefore their data, are not available.
script: /includes/confirmations.php
values: id, title, email, first name, last name, Active Campaign list ID, Mailchimp List ID, Mailerlite group ID, Sendy list ID
Example:
add_action("speakout_signature_confirmed", "update_mailing_list", 10, 9);
function update_mailing_list( $id, $title, $email, $firstName, $lastName, $activecampaignList, $mailchimpList, $mailerliteGroup, $sendyList ){
//create an array including required data
$data = array( 'petitionID' => $id, 'email' => $email, 'listID' => $mailchimpList);
//insert it into database
$wpdb->insert( 'another_table', $data );
}
speakout_goal_updated
Triggered when signature goal is automatically updated. One use would be send a notification so you can check the new goal.
script: /includes/class.signature.php
values: petition id, old goal, increase value
Example:
add_action("speakout_goal_updated", "track_goal", 10, 3);
function track_goal( $id, $oldGoal, $increase ){
mail("administrator@example.com",
"Petition goal auto increased",
"Petition id = " . $id . " old goal = " . $oldGoal . " increased by = " . $increase . " to " . ($oldGoal + $increase)
);
}
Result:
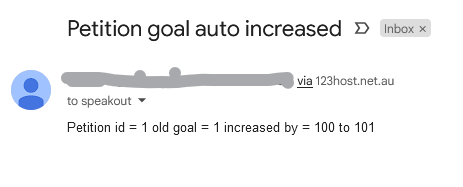
If you have a suggestion for another hook, let me know.